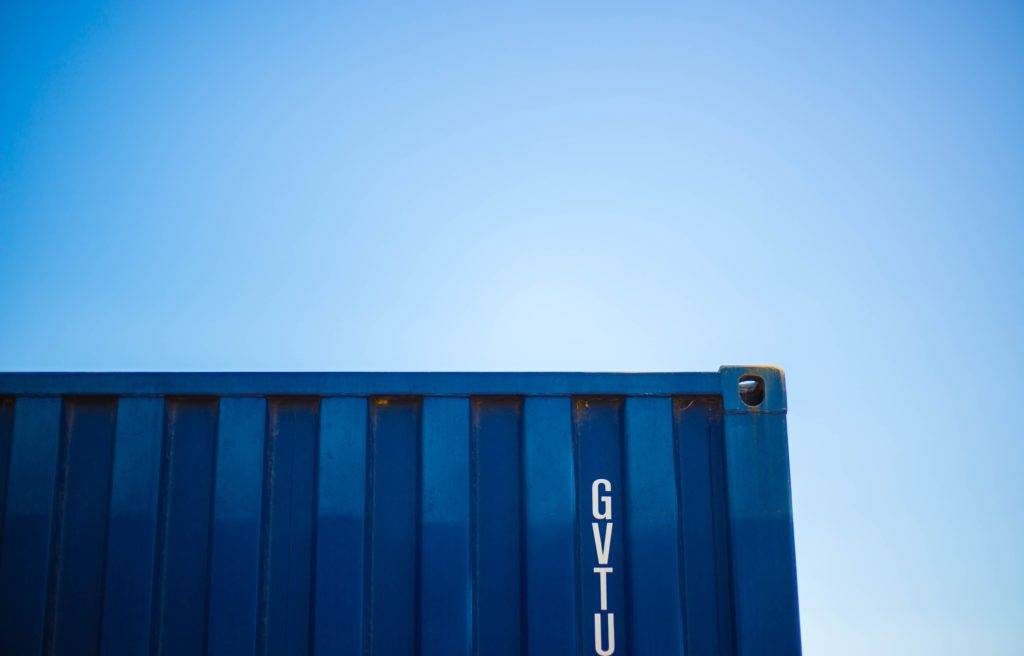
You might need to run ng test or npm install commands inside a docker container for some reason. For example, you might need to do it as a part of continuous integration. So, your CI tool needs to execute these commands. Whatever the reason, in this article, we will look at the way to execute ng and npm commands inside a docker container.
But, first things first, let’s understand some common angular related commands which we will be able to execute inside our docker container.
- npm install
- ng test
- ng build –prod
This command list could go on, but we can make sure to be able to execute some common npm and ng commands inside our docker container.
So, the first thing to do to create a docker image where these commands would be possible to run.
Dockerfile for docker image
Here how our Dockerfile would look like:
FROM trion/ng-cli
USER root
RUN apt-get -y update \
&& apt-get install -y gnupg2 gnupg gnupg1
RUN apt-get install -y wget && apt-get -y update
RUN wget https://dl.google.com/linux/direct/google-chrome-stable_current_amd64.deb
RUN apt install -y ./google-chrome-stable_current_amd64.deb
Let’s elaborate on our Dokcerfile and unerstand what it does.
In the first line, we take a base image as trion/ng-cli. This image would allow us to execute npm and ng commands. But, you might ask why do we need the rest? We need the rest to be able to execute angular test commands: ng test –browsers HeadlessChromeCustom
To execute the ng test commands, you should have a browser installed on your machine. So, by the next lines in Dockerfile, we install chrome browser so that we can run ng test –browsers HeadlessChromeCustom –watch false command inside our container. We will study the params of this command in a bit.
If you don’t want to create the image, it’s available with this tag: yolch/ng-test-build:latest
Unfortunately, only having the docker image is not enough to run ng test command inside the image. We should modify a bit our angular testing configuration.
Angular App Configuration
As a next step, let’s modify our karma.conf.js. Modify the browsers config
browsers: ['Chrome', 'ChromeHeadless', 'ChromeHeadlessCustom'],
customLaunchers: {
ChromeHeadlessCustom: {
base: 'ChromeHeadless',
flags: [
'--no-sandbox',
'--disable-gpu',
'--headless',
'--remote-debugging-port=9222'
]
}
}
Let’s understand this config a bit. We declare that we support three browsers Chrome, ChromeHeadless and ChromeHeadlessCustom. ChromeHeadless is for running chrome without UI. As our container wouldn’t have UI, we should configure ChromeHeadless. Then we customise ChromeHeadless by adding ChromeHeadlessCustom and adding some extra configurations for it.
When we run ng test –browsers HeadlessChromeCustom –watch false it runs HeadlessChrome with custom configs and closes the browser at the end. So, –watch false is for terminating the browser after test cases are executed.
Once this modification is done, we can execute this code inside our docker container and configure our continuous integration tool accordingly.
Now, let’s look how we can do it on jenkins.
Execute ng and npm inside Jenkins
To be able to execute above mentioned commands inside your Jenkins job, you can add the below config in your Jenkinsfile
stage('Run Project') {
agent {
docker {
image 'yolch/ng-test-build:latest'
reuseNode true
}
}
steps {
sh '''
npm install
ng test --browsers ChromeHeadlessCustom --watch false
ng build --prod
'''
}
}
This config will pull the image: yolch/ng-test-build:latest and will execute the commands properly. If you want to pull the image from your custom repository, then you can use the Dockerfile config to generate a new image and push it into your repository.
If you want to modify npm and ng commands as per your needs, you can do it. But remember, you would not be able to run ng test commands without HeadlessChrome config because you can’t start a real chrome browser inside your container.